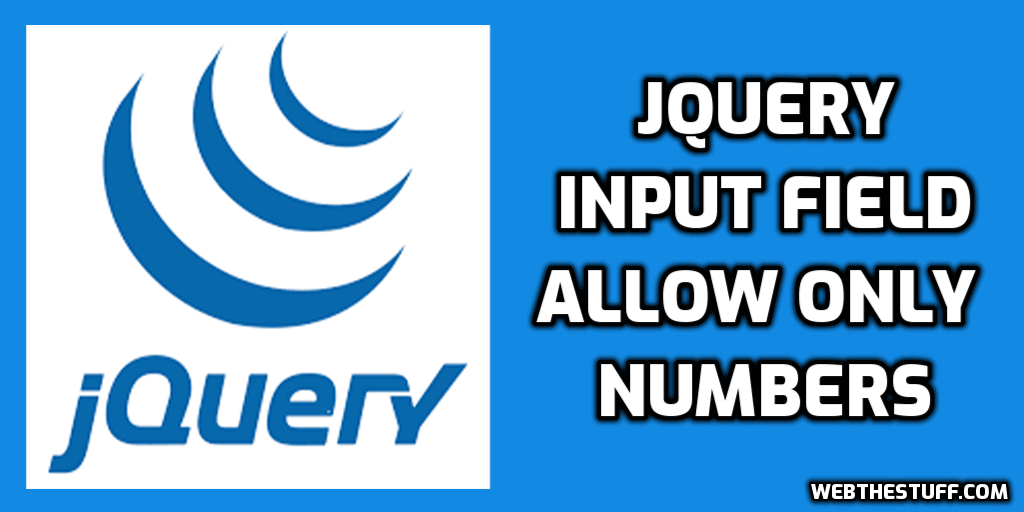
Hi Dev, In this tutorial we will learn how to allow only numbers in textbox using jquery example, This post we implement simple example for input textbox enter only number not allow text and symbol. user key pres and we check this is a number on not if number return true else return false.
If you need to add jquery validation for your textbox like textbox should accept only numbers values on keypress event. you can also use keyup or keypress event to allow only numeric values in textbox using jquery.
We will create a very simple and complete example to allow only number for textbox validation using jquery so you can understand how this example works. Which are as follows.
Example :
<!DOCTYPE html>
<html>
<head>
<title>Allow only numbers in textbox using jquery - phpicoder.com</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<div class="container">
<h1>Allow only numbers in textbox using jquery - phpicoder.com</h1>
<label>Enter Mobile Number:</label>
<input type="text" name="mobile" class="only_numeric" >
<span class="error" style="color: red; display: none">* Input digits (0 - 9)</span>
</div>
<script type="text/javascript">
$(document).ready(function(){
$("body").on("keypress", ".only_numeric", function (event){
var keyCode = event.which ? event.which : event.keyCode
if(!(keyCode >= 48 && keyCode <= 57)){
$(".error").css("display", "inline");
return false;
}else{
$(".error").css("display", "none");
}
});
});
</script>
</body>
</html>
I hope it can help you...