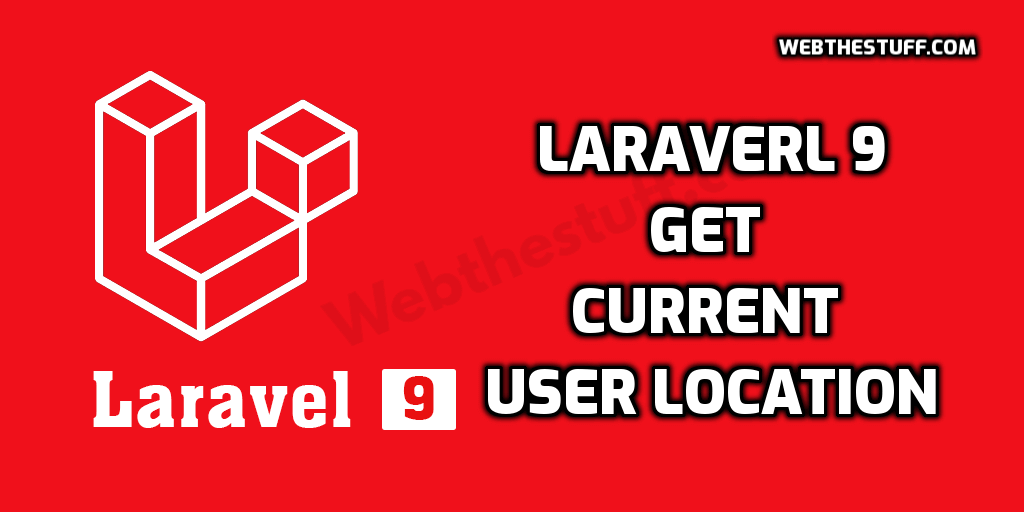
In this tutorial, we will see how to get country name, city name and example of address from ip in laravel9 web app. For your understanding, I have simply explained step by step how to get Laravel 9 location by example. And we will use the stevebauman / location composer package to get the current user location in the laravel application. Which will help us to get the name of the country and the name of the city.
You can learn laravel 9 location country city and state from ip. We'll help you with an example of how to get a country city address from the IP address laravel 9. We will use country name, country code, region code, region name, city name, zip code, latitude and longitude from the IP address. You can just follow the steps below and get the following layout.
Step 1: Create Laravel App
If you already have a newer app, you need to get the latest version of the application using the Belo command, run the following command
composer create-project laravel/laravel blog
Step 2: Install Package
Here, we will install the SteveBowman / Location package to get the current location on the login user.
composer require stevebauman/location
Step 3: Create Route
Here we create a way to get the nearest location from the latitude and long example
Goto routes/web.php file and edit bellow code
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\AreaController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('user-info', [UserController::class, 'index']);
Step 4: Create Controller
Now we need an area controller so run the following command to create a area controller
php artisan make:controller UserController
Goto app/Http/Controllers/UserController.php file and you have users table with location columns.
app/Http/Controllers/UserController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Model\User;
class UserController extends Controller
{
/**
* Get user informations.
*
* @return \Illuminate\Http\Response
*/
public function index(Request $request)
{
/* $ip = $request->ip(); Dynamic IP address */
$ip = '162.159.24.227'; /* Static IP address */
$currentUserInfo = Location::get($ip);
return view('user', compact('currentUserInfo'));
}
}
Step 5: Create View File
here, we need to create blade file for user. so edit bellow code:
Goto resources/views/user.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1>How to Get Current User Location in Laravel Web App- webthestuff.com</h1>
<div class="card">
<div class="card-body">
@if($currentUserInfo)
<h4>IP: {{ $currentUserInfo->ip }}</h4>
<h4>City Name: {{ $currentUserInfo->cityName }}</h4>
<h4>Zip Code: {{ $currentUserInfo->zipCode }}</h4>
<h4>Country Name: {{ $currentUserInfo->countryName }}</h4>
<h4>Country Code: {{ $currentUserInfo->countryCode }}</h4>
<h4>Region Name: {{ $currentUserInfo->regionName }}</h4>
<h4>Region Code: {{ $currentUserInfo->regionCode }}</h4>
<h4>Latitude: {{ $currentUserInfo->latitude }}</h4>
<h4>Longitude: {{ $currentUserInfo->longitude }}</h4>
@endif
</div>
</div>
</div>
</body>
</html>
Step 6: Run Laravel App
This is the last step in which we run Laravel App. Now you have to type the following command and press enter to run the app:
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
Output
Filds | Values |
Ip | 162.159.24.227 |
City Name | Chicago |
Country Name | United States |
Zip Code | 60666 |
Region Name | Illinois |
Region Code | IL |
Latitude | 41.8781 |
Longitude | 87.6298 |
I hope this will help you to understand get country, city name & address from ip address in laravel 9 app