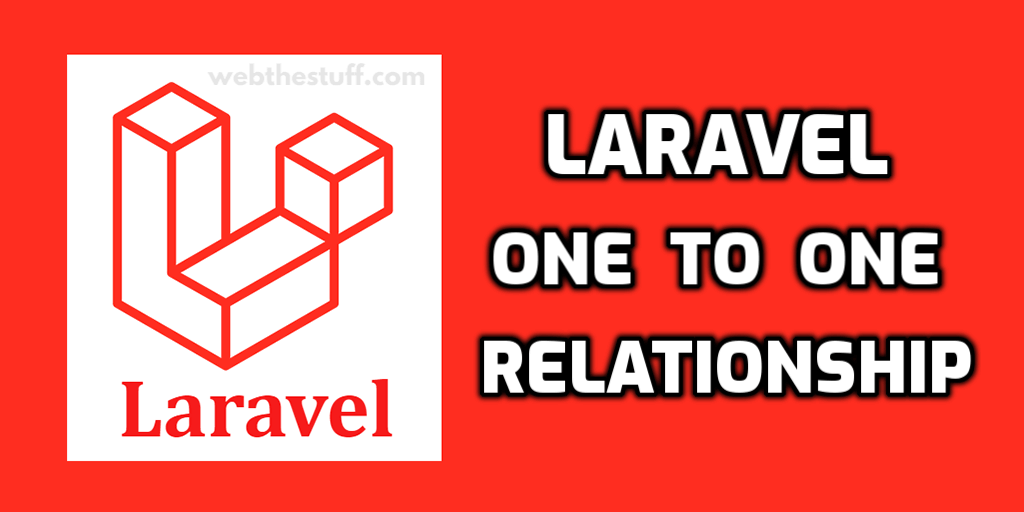
In this tutorial, we will look at how to create a one to one relationship between two models. This is a very basic type of database relationship. For example, a user model is related to an application model.
In this tutorial, we want to explain how Laravel 5, Laravel 6, Laravel 7, Laravel 8 and Laravel 9 form one to one model relationship in applications. The one-to-one model relationship is very simple and basic. You need to make sure that a table contains keys that match other table fields. With that key you will be able to view records from one table to another using one-to-one model relationships.
Laravel offers a eloquent relationship that makes for a powerful query. In Laravel, model classes are used to use eloquent relationships. Laravel eloquent offers simple ways to build common relationships:Laravel offers a chic relationship that makes for a powerful query. In Laravel, model classes are used to use one to one relationships. Laravel eloquent offers simple ways to build common relationships.
Step 1 : Create Migration
In this step, we will create migration. So let us run the following command to create a post table.
php artisan make:migration create_profiles_table
Now next, goto database/migrations/create_profiles_table.php migration file. and edit bellow code.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('profiles', function (Blueprint $table) {
$table->id();
$table->integer('user_id')->unsigned();
$table->integer('mobile');
$table->integer('city');
$table->integer('dist');
$table->integer('pincode');
$table->integer('dob');
$table->boolean('status');
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('profiles');
}
};
Note that we have added user_id (forengn_key) which corresponds to the id (local_key) field of the user table. Below are the profiles migration fields.
Run the migrate command to create tables into database.
php artisan migrate
Step 2 : Create Model
Now, run below command to create Profile model.
php artisan make:model Profile
Then goto app/Models/Profile.php model file and update following code.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
class Profile extends Model
{
use HasFactory;
/**
* Get the user associated with the application.
*/
public function user()
{
return $this->belongsTo(User::class);
}
}
There is already User model in the new Laravel application. So open App\Models\User.php file and edit bellow code
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
use App\Models\Profile;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable;
/**
* Get the application associated with the user.
*/
public function profile()
{
return $this->hasOne(Profile::class);
}
}
Let it be assumed that you have a user_id field in the application table which corresponds to the if field of the user table. If you want to define fields other than user_id in the profile, you can pass the foreign_key as a second argument. Suppose we have a number field corresponding to the ID field of the user table.
public function profile()
{
return $this->hasOne(Profile::class, 'mobile');
}
Step 3 : Create Route
Open routes/web.php file and edit bellow router
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProfileController;
Route::get('/profile', [ProfileController::class, 'index']);
Step 4 : Create Controller
if no have a ProfileController then mack bellow command
php artisan make:controller ProfileController
Now we have to create a new controller as the ProfileController. We will get the user information from them
Find user record that is belongs to profile model
Goto app/Http/Controllers/ProfileController.php and follow below code
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class ProfileController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$profile = User::find(1)->profile;
dd($profile);
}
}
Other Examples
Reverse relationship, a way to access user records.
public function index()
{
$user = Profile::find(1)->user;
dd($user);
}
How to get a single value from a relationship, for example, requires only the user's email.
public function index()
{
$email = Profile::find(1)->user->email;
dd($email);
}
I hope this helps you understand the relationship one by one.