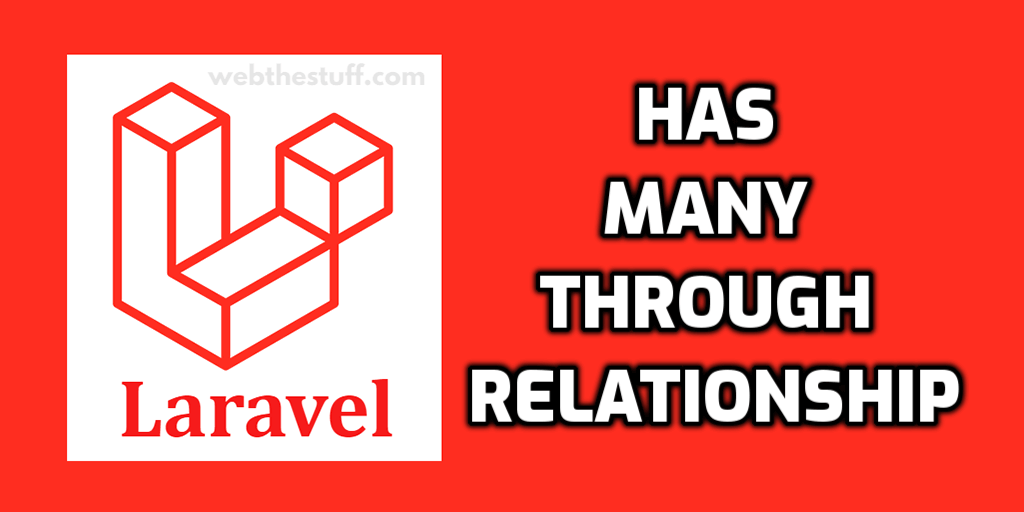
It Has Many Throug relationship providing a convenient way to access distant or intermediate relationships. For example, we have three tables, Users, Posts and Country Table. Now, we want to find posts in that country by user model.
Here we understand how to create multiple through migration relationships with foreign key schema for one to many relationships, in which we will add records and get all the records.
Now we will need "Users", "Post" and "Countries" tables to create the above example. Each table is connected to each other. So first we need to create a database migration, then we need to create a model, then we need to retrieve the record and then we need to know how to create the record.
Step 1 : Create Migrations
Here we will create a migration of "Users", "Posts" and "Countries" M3 table. We will also add a foreign key with users and post table. So follow the code below
go to database/migrations/ and edit users table migration.
Schema::create('users', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->integer('country_id')->unsigned();
$table->rememberToken();
$table->timestamps();
$table->foreign('country_id')->references('id')->on('countries')->onDelete('cascade');
});
go to database/migrations/ and edit posts table migration.
Schema::create('posts', function (Blueprint $table) {
$table->increments('id');
$table->string("name");
$table->integer('user_id')->unsigned();
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
});
go to database/migrations/ and edit countries table migration.
Schema::create('countries', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->timestamps();
});
Step 2 : Create Models with Has Many Through Relationshi
We will model the country below. We will also use "hasManyThrough()" to relate the two models.
Country Model:
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Post;
class Country extends Model
{
public function posts()
{
return $this->hasManyThrough(
Post::class,
User::class,
'country_id', // Foreign key on users table...
'user_id', // Foreign key on posts table...
'id', // Local key on countries table...
'id' // Local key on users table...
);
}
}
Step 3 : Get Items
/**
* get post by particuler country.
*
*/
public function index()
{
$country = Country::find(1);
dd($country->posts);
}
I hope you understand of one to one relationship in larvael app...