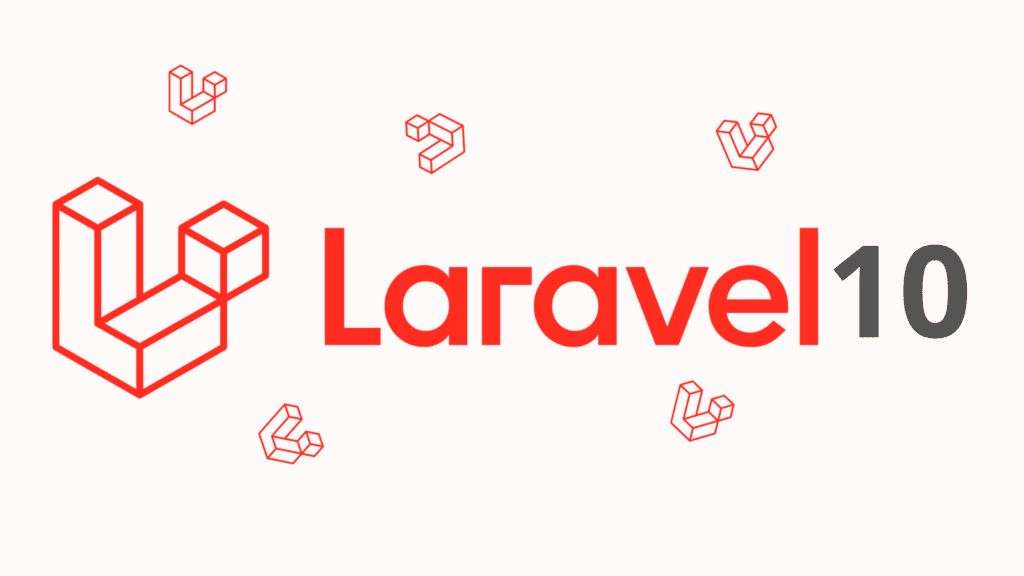
Laravel,
Highcharts JS is a popular JavaScript charting library that is designed to create interactive and visually stunning charts and graphs for web pages. It provides a wide range of charts and graph types such as line chart, area chart, bar chart, column chart, pie chart, scatter chart, and more, which can be customized through a vast number of configuration options.
Step 1: Install Laravel App
composer create-project laravel/laravel example-app
Step 2: Create Route
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\HighchartController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('chart', [HighchartController::class, 'index']);
Step 3: Create Controller
app/Http/Controllers/HighchartController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use DB;
use Illuminate\View\View;
class HighchartController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(): View
{
$users = User::select(DB::raw("COUNT(*) as count"))
->whereYear('created_at', date('Y'))
->groupBy(DB::raw("Month(created_at)"))
->pluck('count');
return view('chart', compact('users'));
}
}
Step 4: Create Blade File:
resources/views/chart.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 10 Highcharts Example - webthestuff.com</title>
</head>
<body>
<h1>Laravel 10 Highcharts Example - webthestuff.com</h1>
<div id="container"></div>
</body>
<script src="https://code.highcharts.com/highcharts.js"></script>
<script type="text/javascript">
var users = {{ Js::from($users) }};
Highcharts.chart('container', {
title: {
text: 'New User Growth, 2022'
},
subtitle: {
text: 'Source: webthestuff.com.com'
},
xAxis: {
categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
},
yAxis: {
title: {
text: 'Number of New Users'
}
},
legend: {
layout: 'vertical',
align: 'right',
verticalAlign: 'middle'
},
plotOptions: {
series: {
allowPointSelect: true
}
},
series: [{
name: 'New Users',
data: users
}],
responsive: {
rules: [{
condition: {
maxWidth: 500
},
chartOptions: {
legend: {
layout: 'horizontal',
align: 'center',
verticalAlign: 'bottom'
}
}
}]
}
});
</script>
</html>
Step 5: Create Dummy Records:
you can create dummy records using laravel tinker command as bellow:
php artisan tinker
User::factory()->count(30)->create()
Run Laravel App:
php artisan serve
Now, Go to web browser, type the given URL and see the output:
http://localhost:8000/chart