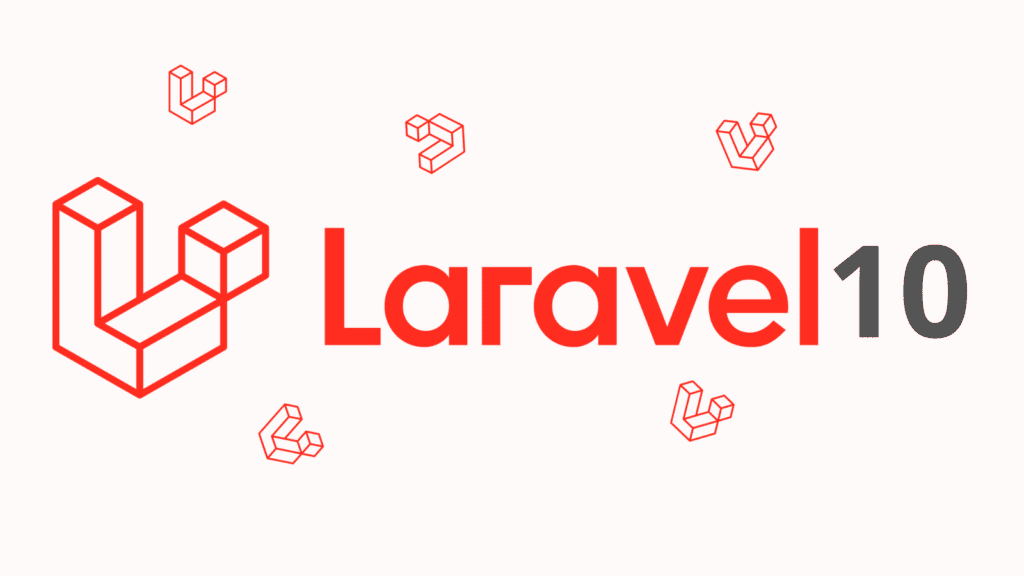
Helper functions allow you to encapsulate commonly used code snippets or utility functions into reusable functions. These functions can be easily called from anywhere in your application, reducing code duplication and promoting reusability.
Step 1: Install Laravel App
composer create-project laravel/laravel example-pagination
Step 2: Create helpers.php File
app/Helpers/helpers.php
<?php
use Carbon\Carbon;
/**
* Write code on Method
*
* @return response()
*/
if (! function_exists('convertYmdToMdy')) {
function convertYmdToMdy($date)
{
return Carbon::createFromFormat('Y-m-d', $date)->format('m-d-Y');
}
}
/**
* Write code on Method
*
* @return response()
*/
if (! function_exists('convertMdyToYmd')) {
function convertMdyToYmd($date)
{
return Carbon::createFromFormat('m-d-Y', $date)->format('Y-m-d');
}
}
Step 3: Register File Path In composer.json File
composer.json
...
"autoload": {
"psr-4": {
"App\\": "app/",
"Database\\Factories\\": "database/factories/",
"Database\\Seeders\\": "database/seeders/"
},
"files": [
"app/Helpers/helpers.php"
]
},
...
After register, we need to run composer auto load command so that will load our helper file.
next run bellow command:
composer dump-autoload
Step 4: Create Route
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('call-helper', function(){
$mdY = convertYmdToMdy('2022-02-12');
var_dump("Converted into 'MDY': " . $mdY);
$ymd = convertMdyToYmd('02-12-2022');
var_dump("Converted into 'YMD': " . $ymd);
});
Run Laravel App:
php artisan serve
Now, Go to web browser, type the given URL and see the output:
http://localhost:8000/call-helper