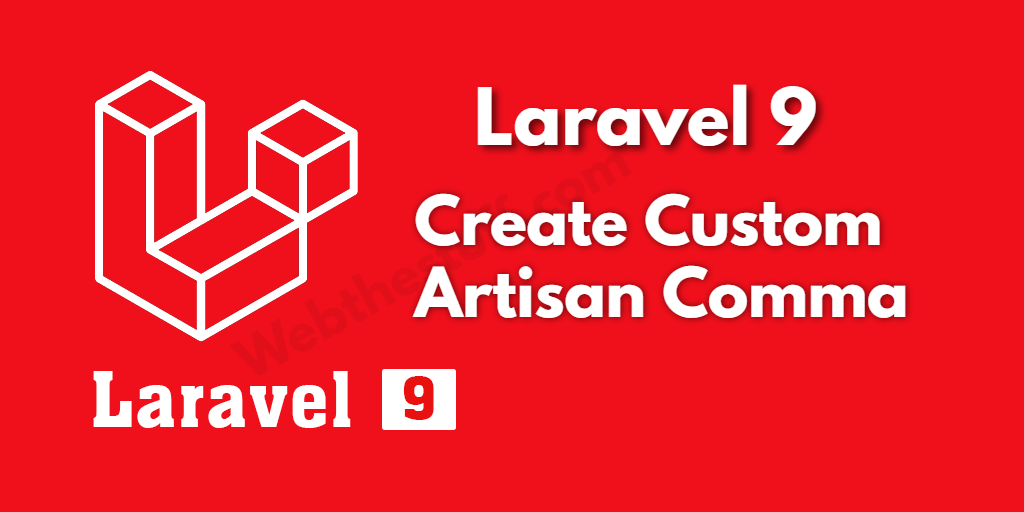
In this tutorials we will share with you how to create custome command in laravel 9 web applictions And we will create an example of laravel 9 create custom artisan.
Thus Laravel provides its own artisan commands to create migrations, models, controllers, etc. But if you want to create your own artisan command for project setup, admin users etc then I will guide you how to create custom artisan command in Laravel application.
In this tutorials, we will create custom "php artisan create:post" command using laravel artisan command. In this command we will pass how many posts to create based on which we will create posts using factory.
so let's follow the below step to create your owl artisan command in laravel app.
Step 1: Create Laravel App
If you already have a newer app, you need to get the latest version of the application using the Belo command, run the following command
composer create-project laravel/laravel web
Step 2: Generate Artisan Command
Here we need to create "CreatePosts" class using below command. Then copy the following code into it. We will add the command name "create-posts".
php artisan make:command CreatePosts
Output :
Goto app/Console/Commands/CreatePosts.php and edit bellow code
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use App\Models\Post;
class CreatePosts extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'create:posts {count}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Create Dummy Posts for your App';
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
$number_of_posts = $this->argument('count');
for ($i = 0; $i < $number_of_posts; $i++) {
Post::factory()->create();
}
return 0;
}
}
Step 3: Use Created Artisan Command
In this step, we will run our custom command
So, let's run following custom command to create multiple posts:
php artisan create:posts 10
You can check in your posts table, it will created records there.
Step 4: Check Command
In this step, you can check your custom command on list as well.
php artisan list
Output :
I hope this will help you to understand Create Custom Artisan Command in Laravel 9 app